Saturday, November 21, 2015
Future growth... AHRS and more channels
After I've completed the basic version, which is using a very small ATMega328 based processor, I'd like to expand.
The next gen processor would be the Teensy LC ($11.65). Why this?
The next gen processor would be the Teensy LC ($11.65). Why this?
- Built in Hardware Serial (hardware I/O buffering)
- Much more powerful 32-bit RISC processor
- More Flash (64kb) and RAM (8kb) to do bigger/faster real-time calculations
- More and better I/O, 13 12-bit Analog inputs
- AHRS / EIS output to a smart phone or tablet via USB. Smart phone MFD/PFD for $60
- More analog inputs. Multiple CHT, EGT, Manifold Pressure, etc
ATAN2Deg (int y, int x)
I'm using a floating point atan2 library, this is a bit expensive for a small processor. Especially when everything I'm doing is in small integers and my output is a small integer degree.
So I did some research, and I found this site with a good approximation, but it had some minor bugs.
Integer Approximation of ATan2
I took that algorithm and created a small C# console app around it.
static int atan2deg(int y, int x)
{
{
var max=Math.Max(y, x);
if (max > 60) // sqrt(2^15/3667)... avoid overflow
x = 60 * x / max;
y = 60 * y / max;
}
if (y==0)
return (x>=0) ? 0 : 180;
if (x==0)
return (y>0) ? 90 : 270;
int aX = Math.Abs(x);
int aY = Math.Abs(y);
int angle = (aY <= aX) ?
(3667 * aX * aY) / (64 * aX * aX + 17 * aY * aY) :
90 - (3667 * aX * aY) / (64 * aY * aY + 17 * aX * aX);
if (x<0)
return (y<0) ? 180 + angle : 180 - angle;
else
return (y<0) ? 360-angle : angle;
}
The algorithm is quite accurate where it worked, but it had bugs;
That said.. it is a very good algorithm
So I did some research, and I found this site with a good approximation, but it had some minor bugs.
Integer Approximation of ATan2
I took that algorithm and created a small C# console app around it.
static int atan2deg(int y, int x)
{
{
var max=Math.Max(y, x);
if (max > 60) // sqrt(2^15/3667)... avoid overflow
x = 60 * x / max;
y = 60 * y / max;
}
if (y==0)
return (x>=0) ? 0 : 180;
if (x==0)
return (y>0) ? 90 : 270;
int aX = Math.Abs(x);
int aY = Math.Abs(y);
int angle = (aY <= aX) ?
(3667 * aX * aY) / (64 * aX * aX + 17 * aY * aY) :
90 - (3667 * aX * aY) / (64 * aY * aY + 17 * aX * aX);
if (x<0)
return (y<0) ? 180 + angle : 180 - angle;
else
return (y<0) ? 360-angle : angle;
}
The algorithm is quite accurate where it worked, but it had bugs;
- It wasn't written in a language I know, but appears similar to basic. There where implied "else" clauses, that I filled-in
- The angle calculation needs to use the abs values of x and y, not the actual values
- The special case X = 0 and Y <= 0 outputs 270 not -90 as this is 001-360 based output
- Scaled input values to ensure we don't overflow 16-bit signed internal math
That said.. it is a very good algorithm
Real progress!
Added to the "Done" list:
Issues:
I destroyed the compass sensor :( by hooking up the 10DoF module backwards which put +5v on an 5v intolerant output pin of just the compass. So I ordered another (cheaper 3-axis compas + BMP180 unit). So yeah, can drop the cost even more. But it won't have 6-axis of acc/gyro.
Left to do:
- Altimeter is working
- ASI is working
- Tach is working (no sensor, just tested pulsing pin 2 line)
- Added an up/down button on digital 4 & 5 INPUT_PULLUP
- Barometer setting page. If you hold the up or down button for a second, it switches to pressure setting mode. if you let go for 2 seconds, it goes back to regular flight mode. Local barometer is permanent, on reboot it will remember your last setting.
- Setup Mode: If you boot with up & down both pressed, it goes into setup mode.
Pressing the down button selects the next parameter; up button sets and stores the current value
Setup menu cycles through the following:
- Airspeed Sensor static pressure
- Airspeed Sensor 6.5" of Water (87kts)
- Oil Temp in Ice Water
- Oil Temp in Boiling water
- Oil Pressure Static
- Oil Pressure RunUp (assumed 80psi)
- Tested the sensors and "calibrate" functions on the ASI. If you blow into it with a straw it goes nicely from 0 to 100+kts. All set via calibration feature built in.
Issues:
I destroyed the compass sensor :( by hooking up the 10DoF module backwards which put +5v on an 5v intolerant output pin of just the compass. So I ordered another (cheaper 3-axis compas + BMP180 unit). So yeah, can drop the cost even more. But it won't have 6-axis of acc/gyro.
Left to do:
- Re-wire to a permanent soldered board (currently just testing on a breadboard)
- Hook up the oil temp sensor, I have it, just needs a voltage splitter resistor and calibration
- Hook up the oil pressure. I don't yet have this sensor, but same voltage splitter.
- Cleanup the code
- refactor into separate files each functions (init, normal, baro, setup)
- Make a smaller integer-math / degrees (vs radians) version of atan2
- Make a smaller integer-math barometer library (very large)
Thursday, November 12, 2015
Display Layout
AIRSPEED HEADING ALTITUDE
OIL PRESSURE RPM OIL TEMP
Status Update:
- Connected the 1602 display
- Laid out the display and it looks clean and straight forward
- Wired up the MPX2010DP(not the recommended part) and op-amp (see diagram below)
- Tested the oil temperature probe which arrived on Tuesday. 1.8kOhm at 70F, dropped to 1kOhm quickly just by pinching it in my warm hands. Simple voltage splitter will work nicely.
- Started on the class that maps analog input to calibrated output. Used on Airspeed, Oil Temp and Oil Pres. Has to store the min/max input values, slope and y-intercept for translating.
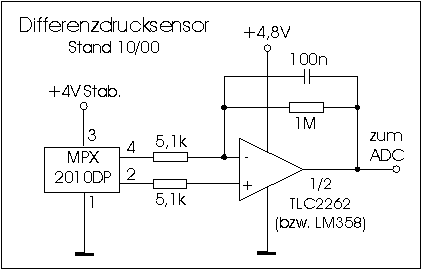
Code Size: 5236 bytes so far.
I do not recommend using the MPX2010DP, instead use an airspeed module suggested in the prior post based on the MPX7002DP. The latter has a very wide voltage response on pressure difference. It does not require an op-amp to condition the signal for the ADC.
I'm still waiting on the 10DoF AHRS board, once that is here, I can make a lot more progress
Tuesday, November 10, 2015
I found this open source EFIS project...
http://sourceforge.net/projects/diy-efis/
The technology is a non-starter... Windows CE. But the concept intrigued me. So I kept digging and found:
https://play.google.com/store/apps/details?id=com.rodriguez.saul.flightgearpfdBasic
This is a more simplistic version intended to be an add-on to an open source flight simulator "Flight Gear", but the application in small experimental aircraft is obvious.
It appears all the open-source EFIS/PFD/MFD/AHRS projects went down the path of "doing it all" themselves. That's a whole lot of propriatary work. Small 7" tablets pack a lot usability in a cheep package most of us already own for other reasons. The one thing a tablet can't do is collect flight data like pressure-derived airspeed, barometric altitude, compass heading, oil temperature, oil pressure and tachometer. In short; this project is exactly what a tablet can't do.
What if we generate AHRS + EIS airdata messages in whatever format an open-sourced-third-party display could render? This would include attitude and SA information flat not feasible on a 16 character by 2 line ASCII LCD display in the project. As most devices have GPS, the table interface would also include additional navigation capabilities, charts and open-source facilities databases and data logging.
Anyway, it's a really cool add on for a $50 AHRS/EIS box... Also, if you switch the processor from A ProMini to a Teensy LC or 3.1; it would be very easy to add up to 14 channels of ADC for 4-CHT/4-EGT/OT/OP/ASI/etc. It could be a good open-source PCB project to simplify the build.
Friday, November 6, 2015
Sport VFR Instrument Project
SportVFR is an DIY electronics instrument system for very simple VFR. I'd like to build a HummelBird some day. This is a hand-prop, no-electrical, 37-hp stick and rudder human kite.
I am a minimalist, in all regards. So I want the simplest possible flight system. Historically this would have meant "steam gauges" or mechanically actuated gauges.
Powered Day VFR, non-electrical flight requires the following gauges (per CFAR part 91);
For flight (nothing can be GPS derived, must be measured)
- Airspeed Indicator
- Non-Sensitive Altimeter - doesn't require adjustment for local barometric pressure
- Magnetic Compass
Engine Instruments
- Tachometer
- Oil Pressure
- Oil Temperature
To purchase all these instrument in mechanical gauges would cost ~$300. While that is far from prohibative, it is nowhere near the most cost effective way anymore. In just the last 5 years small "First Person Vehicles" (aka Drones) have grown out of the R/C community. These vehicles have created a accurate and cheap AHRS (Attitude and Heading Reference System). Add to that the open-source Arduino community making small and powerfull [enough] microprocessors and accessories. All of the above is $51, about the average price of one mechanical instrument.
Here's the BOM:
$1.98 Arduino Pro-Mini (or any compatible arduino-based device)
$1.59 16x2 LCD alph display
$7.54 10-DoF IMU/AHRS, this is 3-axis gyro, accellerometer and compass, with an onboard barometric pressure sensor
$12.56 Differential pressure sensor (airspeed)
$13.98 Oil Pressure Sensor
$9.79 Oil Temperature Sensor
$3.49 Hall-Effect proximity sensor (Tachometer)
Total: $50.93
Display:
Arduino has great libraries for interfacing with the 16x2 LCD display
Sensors:
The sensors come in three flavors, digital-signal, analog-signal and pulse-count.

The AHRS uses a digital signal to transmit the current setting of the device. These signals are digital values. If we wanted to display artificial horizon or turn-bank indicator, we could do so with this device, but it would require additional computations. Fortunately all we really need is the compass and the barometric pressure, both are first-order values that don't require any fancy math or interpreation. Just take the values hit a lookup table and display the scaled result.

The Oil Temperature, Oil Pressure and Airpseed pressures sensor are analog devices. Oil Pressure ranges from 10-180ohms electrical resistence between 0 and 80psi. There are more clevar ways to get high accuracy from this signal, but I just want a rough number and it's much simple to setup a simple voltage splitter using a 500ohm resistor ahead of the sensor and a small capacitor to filter noise. The Oil temperature is very similar but can use a slightly higher resistance (less current) 1.5kOhm. The voltage at the split goes into an Analog Input chanel and can be poled. All sensors will need to be calibrated and scaled, but this can be done using common tools (like 0C ice-water and 100C boiling water for the oil temp).

The final sensor class is pulse-count... this is for the tachometer. It would sound odd that you can count every revolution of a fast moving prop and still do all the stuff above, but actually, it's pretty easy for these little guys. We'll use a low-level processor trick called an "interupt handler" which will wake up every time the tach line rises and incriment a small counter. Then once a second we'll take the count * 60sec and voila; Revs Per Minute.
I/O Summary:
- 2 serial interfaces (LCD and AHRS)
- 3 10-bit Analog Signals
- 1 digital pulse signal
Attacking the coding:
- Create the tach signal interupt
- Setup the LCD display "Hello SimpleVFR"
- Wire the oil temp sensor and read values from it
- Create helper functions for scaling/mapping analog inputs
- Setup the AHRS and read the barometric pressure
- Read the compass
- Start laying out the output to the display from the collected values.
Layout (in roughly PFD order):
[ ASI ] [ HDG ] [ ALT ][v or ^ for vsi]
[ OT ] [ RPM ] [ OP ]
---- EXAMPLE ---
KTS HDG ALT
101 021 3120^
121 2310 63
OT RPM OP
- Add the Oil Pressure and Air pressure sensors and use above calibration/scaling algorithm
- Test in a car to see if airspeed response is linear (acceptable)
- Make values for - ASI hi/lo, OT hi, OP lo and RPM hi - than limits "blink"
- Calibrate all the instruments, I'd like to avoid having a special calibraiton mode, if possible. I should be able to make a version that output raw values and use that to adjust.
Summary:
It's simple and achievable. If anyone wants to follow along and see how it comes out, great. I'm really making this site to get my own ideas out.
Summary:
It's simple and achievable. If anyone wants to follow along and see how it comes out, great. I'm really making this site to get my own ideas out.
Subscribe to:
Posts (Atom)